Java (programming language) : Java is a versatile and widely-used programming language known for its portability, object-oriented principles, and strong community support. Here are some key aspects of Java:
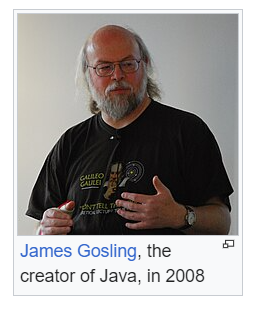
- Object-Oriented: Java is built around the concepts of objects and classes. It encourages modularity and code reuse through inheritance, polymorphism, encapsulation, and abstraction.
- Platform Independence: One of Java’s core features is its platform independence, achieved through the Java Virtual Machine (JVM). Java code is compiled into bytecode, which can run on any system with a JVM, making it “write once, run anywhere.”
- Syntax: Java’s syntax is similar to C++ but with a simpler and more consistent set of features. This includes strong type-checking, automatic memory management through garbage collection, and exception handling.
- Rich Standard Library: Java comes with a comprehensive standard library that includes utilities for networking, data structures, file I/O, and graphical user interfaces (GUIs), among others.
- Concurrency: Java provides built-in support for multi-threading and concurrent programming. This allows developers to write programs that can perform multiple tasks simultaneously.
- Security: Java has several built-in security features, including the sandboxing of applets and the use of the Security Manager, which can help prevent unauthorized access to system resources.
- Popular Use Cases: Java is commonly used for building enterprise-level applications, Android apps, web applications, and large-scale systems. It’s also used in financial services, scientific applications, and big data technologies.
- Development Tools: There are numerous development tools and frameworks available for Java, including integrated development environments (IDEs) like Eclipse, IntelliJ IDEA, and NetBeans, as well as build tools like Maven and Gradle.
If you have any specific questions about Java or need help with a particular aspect of the language, feel free to ask!
Sure! Here’s a more in-depth look at various aspects of Java:
Java Language Features
- Syntax and Structure: Java (programming language)
- Classes and Objects: Java programs are structured around classes and objects. A class is a blueprint for creating objects, and an object is an instance of a class.
- Methods and Variables: Methods define the behavior of objects, while variables store data. Java supports various types of methods including static, instance, and abstract methods.
- Control Flow Statements:
- Conditional Statements: Java uses
if
,else if
,else
, andswitch
for decision-making. - Looping Constructs: Java supports loops such as
for
,while
, anddo-while
for iterative tasks.
- Exception Handling:
- Try-Catch Blocks: Java uses
try
,catch
, andfinally
blocks to handle exceptions and errors in a controlled manner. - Custom Exceptions: Developers can create custom exception classes to handle specific error conditions.
- Generics: Java (programming language)
- Type Safety: Java Generics allow for type-safe collections and methods, reducing the risk of
ClassCastException
and improving code reusability. Java (programming language)
- Annotations:
- Metadata: Annotations provide metadata about the code. They can be used for various purposes, such as generating code, configuring frameworks, or runtime processing.
Java Ecosystem : Java (programming language)
- Java Development Kit (JDK):
- Core Components: The JDK includes the Java Runtime Environment (JRE), which is necessary for running Java applications, and development tools like the compiler (
javac
), Java debugger (jdb
), and JavaDoc tool.
- Integrated Development Environments (IDEs):
- Popular Choices: Eclipse, IntelliJ IDEA, and NetBeans are widely used IDEs that provide tools for coding, debugging, and managing Java projects.
- Build Tools:
- Maven: A build automation tool that uses XML configuration to manage project dependencies and build processes.
- Gradle: A flexible build automation system that uses Groovy or Kotlin DSL for configuration, and is popular for its performance and versatility.
- Frameworks and Libraries:
- Spring Framework: A comprehensive framework for enterprise applications that offers features like dependency injection, aspect-oriented programming, and transaction management.
- Hibernate: An object-relational mapping (ORM) library for managing database interactions.
- Apache Commons: A set of reusable Java components for common programming tasks.
- Java Enterprise Edition (Java EE):
- Servlets and JSP: Technologies for building web applications.
- Java Persistence API (JPA): For managing relational data in Java applications.
- Enterprise JavaBeans (EJB): A server-side component model for building scalable and transactional enterprise applications.
- Java Virtual Machine (JVM):
- Just-In-Time Compilation: The JVM uses JIT compilation to convert bytecode into native machine code at runtime, improving performance.
- Garbage Collection: Automatic memory management is handled by the JVM through garbage collection, which reclaims memory used by objects that are no longer referenced.
Modern Java Trends : Java (programming language)
- Java 8 and Beyond:
- Lambda Expressions: Introduced in Java 8, they provide a concise way to represent anonymous functions.
- Streams API: For functional-style operations on collections, including filtering, mapping, and reduction.
- Optional Class: Helps avoid
null
references and reduceNullPointerException
.
- Recent Updates:
- Java 9+ Features: Includes the Java Module System (JPMS), local-variable type inference (
var
), and pattern matching enhancements. - Java 17: A long-term support (LTS) version with features like sealed classes and improved performance.
- Future Directions:
- Project Loom: Aims to simplify concurrency by introducing lightweight fibers and continuations.
- Project Panama: Focuses on improving the connection between Java and native code.
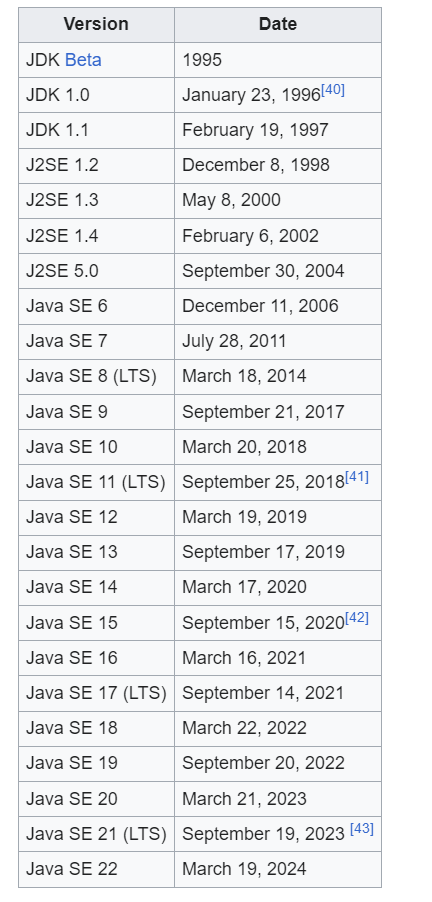
Java continues to evolve, balancing backward compatibility with introducing new features to address modern development challenges. Whether you’re working on enterprise systems, mobile applications, or cloud services, Java remains a robust and relevant choice in the programming landscape.
Certainly! Let’s dive deeper into some additional topics related to Java, including advanced concepts, performance considerations, and real-world applications.
Advanced Java Concepts : Java (programming language)
- Concurrency and Multithreading:
- Threads: Java allows for concurrent execution of threads, which can be managed through the
Thread
class or by implementing theRunnable
interface. - Executors: The
java.util.concurrent
package provides high-level concurrency utilities like theExecutorService
for managing thread pools, tasks, and asynchronous computations. - Synchronization: Ensures that multiple threads do not interfere with each other when accessing shared resources. Java provides synchronized blocks and methods, as well as higher-level constructs like
Lock
andReadWriteLock
.
- Design Patterns:
- Creational Patterns: Such as Singleton, Factory Method, and Abstract Factory, help in object creation mechanisms.
- Structural Patterns: Like Adapter, Decorator, and Proxy, manage object composition and structure.
- Behavioral Patterns: Including Observer, Strategy, and Command, deal with object interactions and responsibilities.
- Java Reflection:
- Runtime Inspection: Reflection allows you to inspect and modify classes, methods, fields, and other elements at runtime. It’s useful for tasks like creating generic frameworks, implementing serialization, or debugging.
- Java Memory Management:
- Heap and Stack Memory: Java manages memory in the heap (for objects) and the stack (for method calls and local variables).
- Garbage Collection Algorithms: Java employs different GC algorithms, such as Serial GC, Parallel GC, and G1 GC, each with its own trade-offs in terms of performance and pause times.
- Java Modules:
- Java Platform Module System (JPMS): Introduced in Java 9, JPMS enables modular programming by defining modules that encapsulate packages and control their visibility. This improves code maintainability and reduces runtime errors.
Performance Considerations
- Profiling and Monitoring:
- Profiling Tools: Tools like VisualVM, JProfiler, and YourKit help in analyzing memory usage, CPU performance, and identifying bottlenecks.
- JVM Monitoring: Tools like JConsole and Java Mission Control provide insights into JVM performance, including garbage collection statistics and thread activity.
- Optimization Techniques:
- Code Optimization: Write efficient algorithms and use data structures suited to your needs. Avoid premature optimization; profile and identify real performance issues first.
- JVM Tuning: Adjust JVM parameters (e.g., heap size, GC settings) based on your application’s requirements and workload characteristics.
- Concurrency Optimization: Use concurrent data structures and avoid unnecessary synchronization to improve multi-threaded performance.
Real-World Applications of Java
- Enterprise Applications:
- Java EE (Jakarta EE): Enterprise applications often use Java EE technologies for building scalable, distributed, and secure applications. Examples include ERP systems, CRM systems, and large-scale web applications.
- Web Development:
- Servlets and JSP: Java Servlets and JavaServer Pages (JSP) are foundational technologies for creating web applications. Modern frameworks like Spring MVC build on these technologies to simplify development.
- Frameworks: Spring Boot is widely used for creating microservices and web applications, providing embedded servers and easy configuration.
- Mobile Development:
- Android Development: Java was the primary language for Android development before Kotlin became a popular alternative. Many Android applications are still written in Java, and the Android SDK provides extensive libraries and APIs.
- Big Data:
- Apache Hadoop: Java is used extensively in the Hadoop ecosystem for processing and analyzing large datasets.
- Apache Kafka: A distributed streaming platform written in Java, used for building real-time data pipelines and streaming applications.
- Financial Services:
- Trading Systems: Java (programming language) High-frequency trading systems and financial analytics often leverage Java for its performance and reliability.
- Banking Applications: Java’s robustness and scalability make it a popular choice for banking and financial applications.
- Embedded Systems:
- Java ME (Micro Edition): Java (programming language) Though less common today, Java ME was used in embedded systems and mobile devices, offering a lightweight platform for resource-constrained environments.
Community and Ecosystem
- Open Source Projects:
- Apache Software Foundation: Java (programming language) Provides a wide range of open-source Java projects, including libraries and frameworks.
- Eclipse Foundation: Home to many Java-related projects, including the Eclipse IDE and various Java development tools.
- Community Support: Java (programming language)
- Forums and Q&A Sites: Websites like Stack Overflow and Reddit have active Java communities where developers share knowledge and help each other.
- Java User Groups (JUGs): Local groups and meetups offer networking opportunities, presentations, and discussions on Java-related topics.
Java’s extensive ecosystem and community support ensure that it remains a powerful and relevant choice for a wide range of applications. Whether you’re working on a new project or maintaining an existing system, understanding these advanced concepts and leveraging best practices will help you make the most of Java’s capabilities.
Certainly! Let’s explore further aspects of Java, including its role in emerging technologies, best practices for development, and resources for continuous learning.
Java in Emerging Technologies
- Cloud Computing:
- Cloud Platforms: Java is widely used in cloud environments, with major cloud providers like AWS, Google Cloud, and Microsoft Azure offering robust support for Java applications. Services such as AWS Lambda and Google App Engine provide environments for deploying Java applications in the cloud.
- Microservices: Java frameworks like Spring Boot and Micronaut simplify the development and deployment of microservices, which are often used in cloud-native applications.
- Machine Learning and Data Science:
- Libraries and Frameworks: Java libraries like DeepLearning4j and Weka provide tools for machine learning and data analysis. While Python is more dominant in this field, Java’s performance and scalability make it suitable for production-grade ML systems.
- Integration: Java can be used to integrate with other machine learning tools and platforms, such as TensorFlow and Apache Spark, often through APIs or Java bindings.
- Internet of Things (IoT): Java (programming language)
- Embedded Systems: Java ME and Java SE Embedded are used in various IoT applications, providing a platform for developing software for connected devices.
- Protocols and Frameworks: Java supports IoT protocols like MQTT and frameworks like Eclipse Kura, enabling the development of scalable and efficient IoT solutions.
- Blockchain: Java (programming language)
- Blockchain Platforms: Java (programming language) Java is used in blockchain development, with platforms like Hyperledger Fabric providing Java SDKs for building and managing blockchain networks.
- Smart Contracts: Java can be used to develop smart contracts and blockchain applications, leveraging its performance and security features.
Best Practices for Java Development
- Code Quality and Maintainability:
- Coding Standards: Follow Java coding conventions and best practices to improve readability and maintainability. Tools like Checkstyle and PMD can help enforce coding standards.
- Documentation: Use JavaDoc to document your code, making it easier for others (and yourself) to understand and use your classes and methods.
- Testing:
- Unit Testing: Use testing frameworks like JUnit and TestNG to write and run unit tests, ensuring that individual components of your application work correctly.
- Integration Testing: Tools like Arquillian and Spring Test can be used for integration testing, verifying that different parts of your application work together as expected.
- Mocking: Use libraries like Mockito for creating mock objects in your tests, allowing you to isolate and test components without dependencies on external systems.
- Performance Optimization:
- Profiling and Benchmarking: Regularly profile and benchmark your application to identify and address performance bottlenecks.
- Efficient Data Structures: Choose appropriate data structures for your use case to optimize performance and memory usage.
- Concurrency: Java (programming language)
- Thread Safety: Ensure that your code is thread-safe when working with shared resources. Use concurrent data structures and synchronization mechanisms as needed.
- Avoiding Deadlocks: Be cautious of potential deadlocks by carefully managing locks and avoiding circular dependencies.
- Security:
- Input Validation: Always validate user input to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS).
- Secure Coding Practices: Follow secure coding guidelines and use tools to scan for vulnerabilities in your code.
Resources for Continuous Learning
- Online Courses and Tutorials:
- MOOCs: Platforms like Coursera, edX, and Udemy offer courses on Java programming, ranging from beginner to advanced levels.
- Interactive Tutorials: Websites like Codecademy and Pluralsight provide interactive Java programming tutorials and exercises.
- Books:
- “Effective Java” by Joshua Bloch: A highly recommended book that provides practical advice on writing high-quality Java code.
- “Java: The Complete Reference” by Herbert Schildt: A comprehensive guide to Java programming, covering basic to advanced topics.
- Documentation and Specifications:
- Oracle’s Java Documentation: Official documentation for Java SE, including API references, guides, and tutorials.
- Java Language Specification (JLS): The formal specification of the Java programming language, providing detailed information on language features and behavior.
- Community and Forums:
- Stack Overflow: A valuable resource for asking questions, finding answers, and participating in discussions about Java programming.
- Reddit: Subreddits like r/java and r/learnjava offer discussions, advice, and resources related to Java development.
- Conferences and Meetups:
- JavaOne: An annual conference focused on Java technologies, featuring sessions from industry experts and opportunities for networking.
- Local Meetups: Participating in local Java user groups or meetups can provide opportunities for learning, networking, and staying updated on Java trends.
- Blogs and News Sites:
- JavaWorld and DZone: Websites that publish articles, tutorials, and news related to Java development.
- Medium and Dev.to: Platforms where developers share insights, tips, and experiences related to Java and software development.
By exploring these resources and embracing best practices, you can stay updated with the latest developments in Java and continuously improve your skills as a Java developer. Whether you are working on enterprise applications, exploring new technologies, or contributing to open-source projects, Java remains a powerful and relevant language in the evolving landscape of software development.